Mastering JavaScript Programming Paradigms: OOP vs Functional Programming
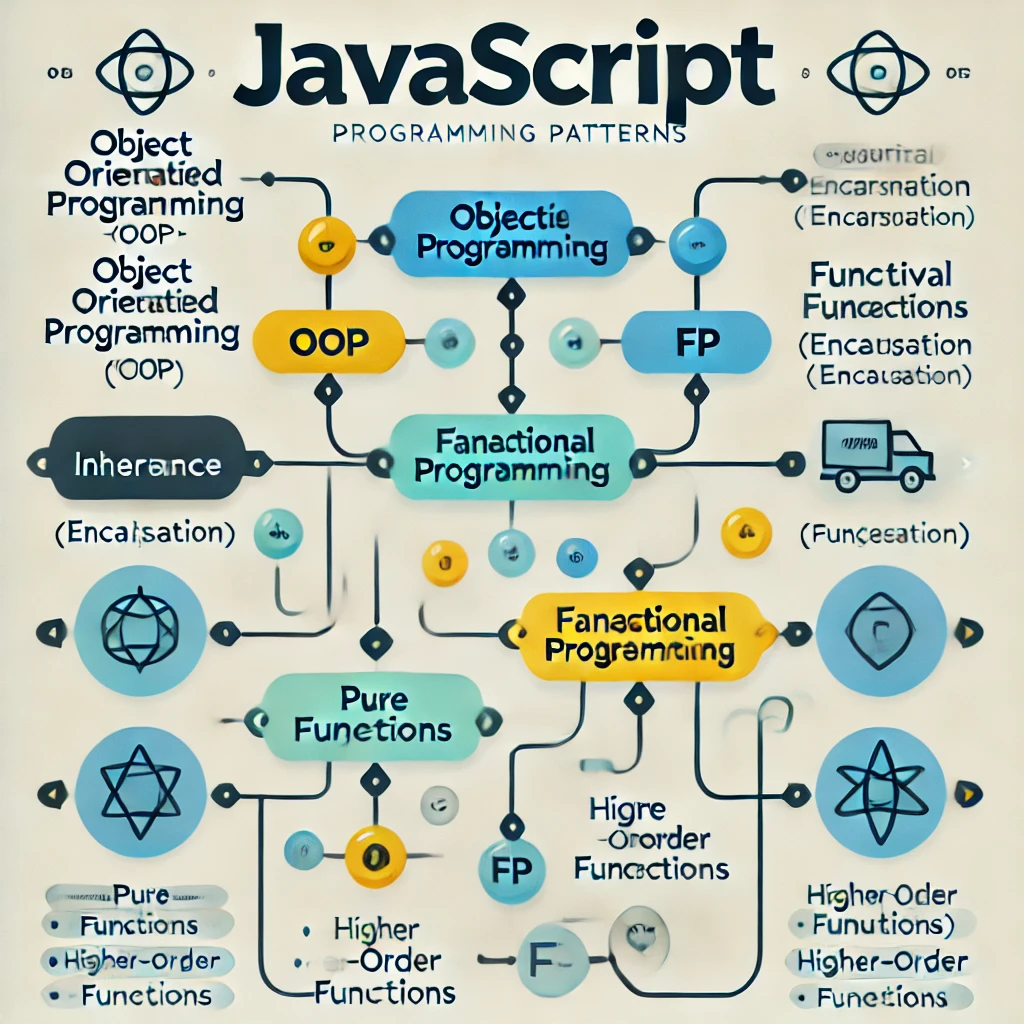
Delve deep into the world of JavaScript programming paradigms, comparing Object-Oriented Programming (OOP) with Functional Programming (FP). Learn the key patterns, concepts, and understand when to use each paradigm, complete with real-world examples and in-depth analysis.
JavaScript is a versatile language that offers different ways to solve problems, but two of the most prominent approaches are Object-Oriented Programming (OOP) and Functional Programming (FP). Each paradigm has its unique way of organizing and thinking about code, and both have their strengths and weaknesses.
Whether you’re building a game, a web app, or processing data, understanding these paradigms will help you write cleaner, more efficient, and maintainable code. This post will guide you through an in-depth exploration of OOP and FP in JavaScript, showing you the patterns, pros, and cons of each approach.
Object-Oriented Programming (OOP): Structuring Code Around Objects
OOP is like building with LEGO blocks – you create objects (blocks) that have both properties (characteristics) and methods (actions). This makes it easier to model real-world concepts, making your code more intuitive and easier to manage, especially as it scales.
Key Concepts of OOP
1. Classes and Objects
In OOP, a class is a blueprint for creating objects. Objects are instances of these classes.
Example
// Creating a class for a simple Car
class Car {
constructor(make, model) {
this.make = make;
this.model = model;
}
drive() {
console.log(`${this.make} ${this.model} is driving`);
}
}
// Creating objects (instances) from the class
const myCar = new Car("Toyota", "Corolla");
myCar.drive(); // Output: "Toyota Corolla is driving"
Classes make it easy to create multiple objects with similar characteristics and behaviors, such as cars in our example.
2. Inheritance
Inheritance allows one class to extend another, reusing its properties and methods. It’s like children inheriting features from their parents.
Example
// Parent class
class Animal {
speak() {
console.log("The animal makes a sound");
}
}
// Child class that inherits from Animal
class Dog extends Animal {
speak() {
console.log("The dog barks");
}
}
const dog = new Dog();
dog.speak(); // Output: "The dog barks"
Pros:
- Reusability of code
- Simplifies complex structures
Cons:
- Can lead to tightly coupled code
- Overuse can make debugging difficult
Common OOP Patterns in JavaScript
The Singleton Pattern
This pattern ensures there is only one instance of a class throughout your application.
class Database {
constructor() {
if (Database.instance) return Database.instance;
Database.instance = this;
}
}
const db1 = new Database();
const db2 = new Database();
console.log(db1 === db2); // Output: true
Pros and Cons of OOP
Pros | Cons |
---|---|
Models real-world problems naturally | Can become complicated with large hierarchies |
Encourages code reuse and modularity | Can lead to tightly coupled code |
Great for projects with complex state | Requires more boilerplate code |
Functional Programming (FP): Treating Code as a Series of Transformations
FP treats computation as the evaluation of mathematical functions, avoiding changing state and mutable data. It emphasizes writing functions that are predictable and reusable.
Key Concepts of FP
1. Pure Functions
A pure function always produces the same output for the same input and has no side effects (it doesn’t change anything outside of itself).
Example
// Pure function example
function add(a, b) {
return a + b;
}
Pure functions are easier to test and debug since they’re predictable.
2. Immutability
In FP, data is never changed directly. Instead, you create new versions of data.
Example
// Immutable operation
const numbers = [1, 2, 3];
const newNumbers = [...numbers, 4];
console.log(numbers); // Output: [1, 2, 3]
console.log(newNumbers); // Output: [1, 2, 3, 4]
3. Higher-Order Functions
These are functions that take other functions as arguments or return them.
Example
// Higher-order function example
function applyOperation(arr, operation) {
return arr.map(operation);
}
const result = applyOperation([1, 2, 3], (num) => num * 2);
console.log(result); // Output: [2, 4, 6]
Common FP Patterns in JavaScript
Function Composition
Combining multiple simple functions to build more complex ones.
const multiplyBy2 = (x) => x * 2;
const add3 = (x) => x + 3;
const multiplyAndAdd = (x) => add3(multiplyBy2(x));
console.log(multiplyAndAdd(5)); // Output: 13
Pros and Cons of FP
Pros | Cons |
---|---|
Predictable, testable functions | Can be less intuitive for complex state changes |
Reduces side effects | Can lead to more complex function compositions |
Emphasizes code reusability | Sometimes less efficient with memory usage due to immutability |
Comparing OOP and FP
Aspect | OOP | FP |
---|---|---|
Data Handling | Mutable | Immutable |
Code Structure | Based on objects and methods | Based on functions and transformations |
Best Use Cases | Complex UI, games, apps with state | Data processing, calculations, stateless tasks |
Final Thoughts
Both OOP and FP have their places in JavaScript development. While OOP is excellent for modeling real-world entities and relationships, FP shines when you want predictable, reusable, and testable code. The beauty of JavaScript is that you don’t have to choose one or the other – you can mix and match these paradigms to suit your project’s needs.
By understanding these paradigms and patterns, you’ll have a more powerful toolkit for writing maintainable, efficient, and scalable JavaScript code.