Mastering AWS: Essential Services Every Programmer Should Know
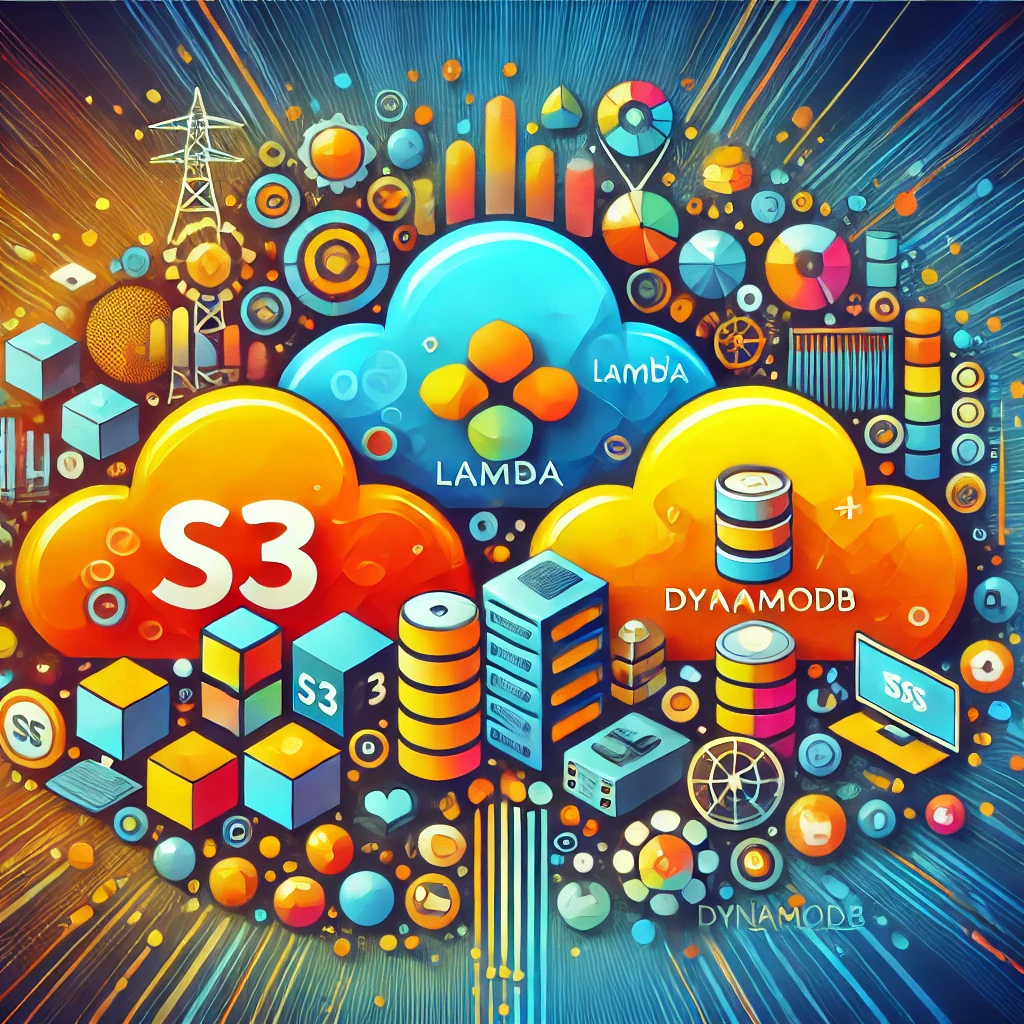
AWS offers a vast array of services, but some are more fundamental to daily programming than others. This blog explores the essential AWS services every developer should learn, from S3 to Lambda, with real-world examples and practical use cases.
Amazon Web Services (AWS) has redefined the way we build, deploy, and manage applications. With its sheer number of services, choosing the right ones for your project can be overwhelming. However, mastering a core set of services can significantly enhance your development workflow. In this post, I’ll focus on the most essential AWS services that every programmer should learn. These services form the backbone of any cloud-based project, from hosting websites to deploying microservices.
Let’s break down these services with in-depth explanations and real-world programming tasks.
Amazon S3 (Simple Storage Service)
What It Is:
Amazon S3 is a highly scalable and secure object storage service. It allows you to store and retrieve unlimited data in the cloud, making it perfect for static content like images, videos, backups, and even hosting static websites.
Why You Should Use It:
S3 is critical for projects involving file storage, backups, or media handling. Its durability (99.999999999%) and seamless integration with other AWS services make it the go-to solution for storing large datasets or serving static files.
Example: Hosting a Static Website on S3
If you’ve developed a static website, like a portfolio using Astro.js, you can host it on S3. With a few commands, you’ll have a cost-effective, highly available static site.
# Sync local files to an S3 bucket
aws s3 sync ./my-website s3://my-static-site-bucket --acl public-read
# Enable static website hosting on the bucket
aws s3 website s3://my-static-site-bucket/ --index-document index.html
With S3, there’s no need for a traditional web server. You can also set up automatic backups or serve content through CloudFront for even better performance and security.
Amazon DynamoDB
What It Is:
DynamoDB is a fully managed, key-value and document NoSQL database service. It’s designed for fast and flexible data models, with consistent low-latency performance at scale. It automatically scales to meet your application’s demand and integrates easily with other AWS services.
Why You Should Use It:
DynamoDB is essential for applications that require real-time data processing, like gaming leaderboards, session management, or IoT data tracking. Its ability to handle high throughput without infrastructure management makes it a great choice for mission-critical apps.
Example: Building a Todo List API
Consider a serverless application where you store user tasks in DynamoDB. Each task is stored as an item in a DynamoDB table:
const AWS = require("aws-sdk");
const dynamoDb = new AWS.DynamoDB.DocumentClient();
exports.handler = async (event) => {
const params = {
TableName: "Todos",
Item: {
id: event.id,
task: event.task,
completed: event.completed,
},
};
await dynamoDb.put(params).promise();
return { statusCode: 200, body: JSON.stringify("Task added!") };
};
DynamoDB’s auto-scaling, on-demand capacity, and integrated caching (via DAX) make it the perfect choice for highly responsive APIs, especially those built with a serverless architecture like AWS Lambda.
AWS IAM (Identity and Access Management)
What It Is:
IAM is the service responsible for managing access to AWS resources. It allows you to define who (authentication) can access which services and resources (authorization). You can create users, groups, roles, and assign permissions to ensure proper access control.
Why You Should Use It:
Proper IAM management is critical for securing your AWS environment. By using the principle of least privilege, you minimize the risk of security breaches. IAM policies ensure that every user, application, or service has just the access it needs.
Example: Creating a User with Limited S3 Access
Let’s say you’re working on a project where a team member only needs read access to certain S3 buckets.
# Create an IAM user
aws iam create-user --user-name ReadOnlyUser
# Attach a read-only S3 policy to the user
aws iam attach-user-policy --user-name ReadOnlyUser --policy-arn arn:aws:iam::aws:policy/AmazonS3ReadOnlyAccess
IAM also allows you to set up multi-factor authentication (MFA), audit access with CloudTrail, and use roles to manage permissions for applications securely.
AWS Systems Manager
What It Is:
AWS Systems Manager provides a unified interface for managing your AWS infrastructure. It offers automation tools for patching, configuration, and operations data, and it helps streamline system management across multiple AWS resources.
Why You Should Use It:
Systems Manager is essential for managing large-scale environments with multiple EC2 instances, RDS databases, or even on-premise servers. It enables you to run commands remotely, automate maintenance, and monitor system performance.
Example: Running a Command Across Multiple EC2 Instances
If you need to patch or update multiple EC2 instances, you can use Systems Manager to execute the commands remotely.
aws ssm send-command --document-name "AWS-RunPatchBaseline" \
--targets "Key=instanceIds,Values=i-0abcd1234efgh5678"
With Session Manager, you can also securely access instances without needing SSH or bastion hosts, reducing attack surface and improving security.
AWS CodeBuild
What It Is:
CodeBuild is a fully managed build service that compiles your source code, runs tests, and produces deployable artifacts. It’s fully scalable and integrates with other AWS services like CodePipeline and CodeCommit for seamless CI/CD processes.
Why You Should Use It:
For any application with continuous integration needs, CodeBuild is crucial. It supports various programming languages, custom build environments, and scales automatically depending on the size of the build.
Example: CI/CD for a Node.js Application
Here’s an example of a basic buildspec.yml
file for running tests and packaging a Node.js app.
version: 0.2
phases:
install:
commands:
- npm install
build:
commands:
- npm test
artifacts:
files:
- "**/*"
With CodeBuild, you can automate the testing and building of your application, which ensures higher code quality and faster iteration times.
AWS CodePipeline
What It Is:
AWS CodePipeline is a fully managed continuous delivery service that helps you automate your release process. It integrates with CodeBuild, Lambda, S3, and third-party tools like GitHub, Jenkins, and Bitbucket.
Why You Should Use It:
CodePipeline automates the build-test-deploy process, helping to reduce human error, improve consistency, and speed up the deployment process.
Example: Automating Static Website Deployment to S3
CodePipeline can be used to automate deployments whenever you push changes to a GitHub repo.
Pipeline:
Source:
- GitHub
Build:
- AWS CodeBuild
Deploy:
- S3
With CodePipeline, you can create complex, automated pipelines that ensure changes are built, tested, and deployed as soon as they’re committed, keeping your team productive.
AWS Lambda
What It Is:
AWS Lambda is a serverless compute service that runs code in response to events. It’s perfect for event-driven applications where you want to execute code in response to triggers like HTTP requests, file uploads, or scheduled tasks.
Why You Should Use It:
Lambda allows you to build scalable applications without managing servers. You only pay for the compute time consumed by your code, making it cost-efficient for small, infrequent tasks or heavy burst traffic applications.
Example: Processing Images Upon Upload
When an image is uploaded to an S3 bucket, Lambda can be triggered to automatically resize the image:
exports.handler = async (event) => {
const s3 = new AWS.S3();
const params = { Bucket: "my-bucket", Key: event.key };
// Image resizing logic here...
await s3.putObject(params).promise();
return { statusCode: 200, body: "Image processed!" };
};
This “pay-as-you-go” model is highly efficient for event-driven architectures where functions are only triggered as needed.
AWS CodeArtifact
What It Is:
AWS CodeArtifact is a fully managed artifact repository for software packages. It supports package managers like npm, Maven, and pip, making it ideal for sharing libraries and dependencies across your team or organization.
Why You Should Use It:
Managing custom libraries and dependencies is easier and more secure with CodeArtifact. It eliminates the need for third-party repositories, and you can integrate it with your CI/CD pipeline for streamlined development workflows.
Example: Publishing a Custom npm Package
You can easily configure npm to use CodeArtifact for storing and sharing your custom packages.
# Set up npm to use CodeArtifact
aws codeartifact login --tool npm --repository my-repo --
domain my-domain
# Publish your package
npm publish
CodeArtifact ensures your package dependencies are available, reliable, and versioned appropriately.
AWS Amplify
What It Is:
AWS Amplify is a comprehensive platform for building scalable, serverless web and mobile applications. It simplifies backend management and front-end deployment, and includes built-in features like authentication, storage, and APIs.
Why You Should Use It:
Amplify makes it incredibly easy to build full-stack applications. It abstracts much of the backend complexity, allowing you to focus on front-end development and user experience. It integrates seamlessly with services like Cognito for user authentication and S3 for storage.
Example: Deploying a Full-Stack React App
With Amplify, deploying a React app with an API backend becomes straightforward.
# Initialize your Amplify project
amplify init
# Add hosting and backend resources
amplify add hosting
amplify add api
Amplify can handle deployment, authentication, API management, and storage, significantly reducing your operational overhead.