Developing Good Programming Habits
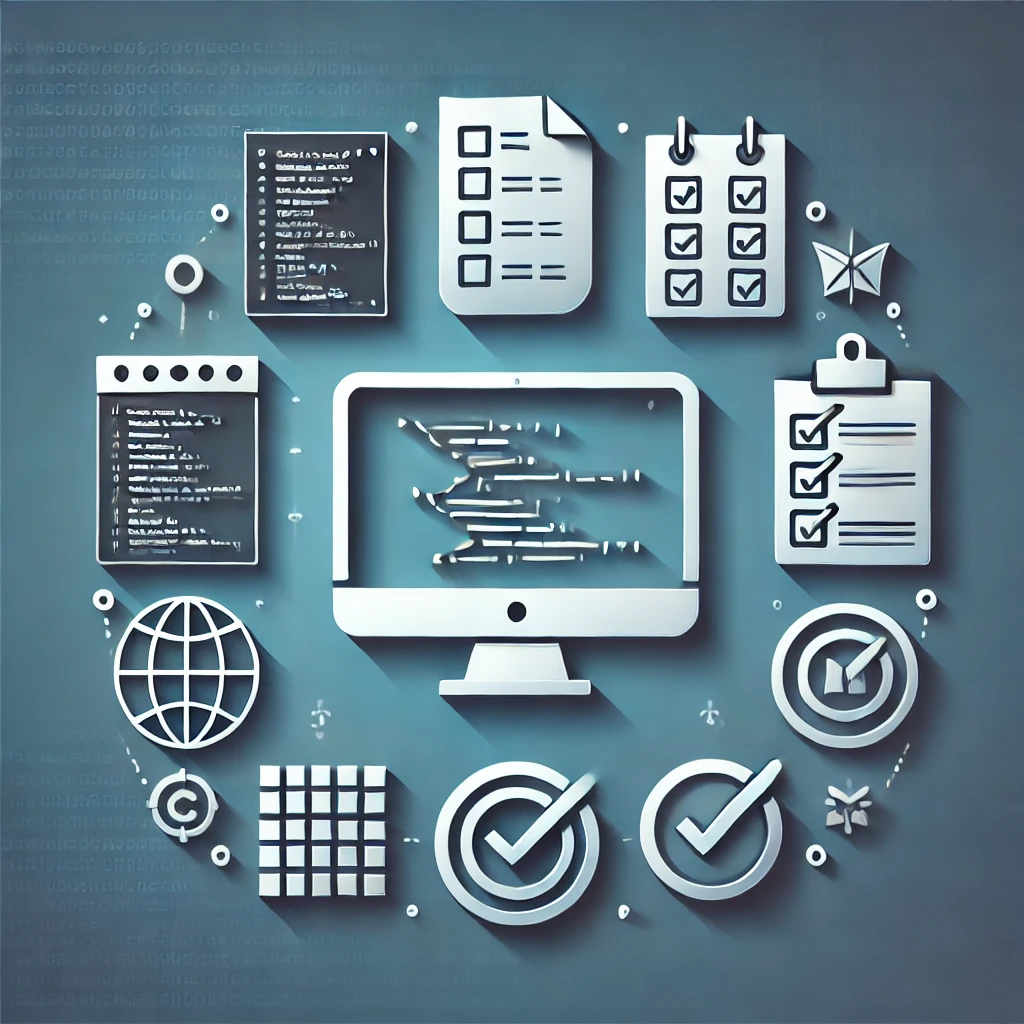
Cultivating good programming habits is essential for improving code quality, boosting productivity, and maintaining a healthy work-life balance. Learn how building these habits can transform your development career.
Programming isn’t just about solving problems; it’s about solving them efficiently, sustainably, and with a mindset that enables long-term growth. Whether you’re a junior developer just starting out or a senior programmer managing a large-scale system, the habits you form will play a crucial role in shaping your journey. Here, we’ll explore the key habits that have helped me become a better developer, with real-world scenarios and practical examples you can apply to your daily coding life.
Writing Clean, Self-Explanatory Code
The old adage, “Code is read more often than it’s written,” couldn’t be more accurate. When you’re writing code, you’re not just writing for yourself in the moment; you’re writing for future you, your team, and anyone who will ever touch that codebase. Clean, self-explanatory code can make debugging, scaling, and collaboration easier for everyone.
Example: Meaningful Variable Names and Comments
Think about the last time you revisited a piece of code you wrote months ago. Did it take you a while to figure out what you were thinking? Now, imagine how hard it must be for someone else to understand it.
// Confusing and unclear code
let x = 7200;
if (userAuth) {
doStuff();
}
// Clear and meaningful code
const SECONDS_IN_TWO_HOURS = 7200;
const isUserAuthenticated = userAuth;
if (isUserAuthenticated) {
processUserLogin();
}
In a real-world scenario, imagine you’re working on a payment system. If you name variables vaguely, like x
or y
, debugging a failed transaction months later will be a nightmare. But by using meaningful names like SECONDS_IN_TWO_HOURS
, future debugging becomes faster and far less stressful.
It’s also helpful to leave concise comments where the intent of your code might not be obvious. Just don’t overdo it—your code should ideally explain itself.
Consistency in Code Structure
One of the things that distinguish senior developers from juniors is their attention to consistency. Consistent code makes it easier to navigate large codebases, allows for smooth collaboration, and makes refactoring much simpler.
Example: Consistent Function Naming Conventions
When you name functions, pick a naming convention and stick with it across the entire codebase. Consistency allows both you and others to anticipate the structure of the code.
// Inconsistent naming conventions
function addTask(task) {}
function remove_task(id) {}
function UpdateTasks(tasks) {}
// Consistent naming conventions
function addTask(task) {}
function removeTask(id) {}
function updateTasks(tasks) {}
A real-world scenario? You’ve inherited a project from a colleague, and the function naming is all over the place. It’s going to take you significantly longer to understand what each function does if the naming isn’t uniform. Imagine working in a collaborative environment where ten people contribute to the same project—consistent conventions will save you hours of mental gymnastics.
Breaking Down Problems
When faced with a large, daunting task, it’s natural to feel overwhelmed. One of the best habits you can develop is breaking big problems into smaller, manageable pieces. This not only makes the task feel more achievable but also allows you to focus on solving one problem at a time.
Example: Refactoring a Legacy Codebase
Let’s say you’ve been assigned the task of refactoring a messy, sprawling legacy codebase. Instead of trying to tackle it all at once, break it down into smaller tasks. Start by isolating the core functionality, identifying duplicated code, and working module by module.
// Instead of trying to fix everything at once
function processOrder(order) {
// Complex business logic, database calls, and more
}
// Break it down into smaller functions
function validateOrder(order) {
// Validation logic
}
function applyDiscounts(order) {
// Discount logic
}
function finalizeOrder(order) {
// Process final steps
}
function processOrder(order) {
if (!validateOrder(order)) return;
applyDiscounts(order);
finalizeOrder(order);
}
This modular approach makes your refactoring project more manageable and allows you to test and debug each part of the process in isolation. In a real-world job, this habit can be a life-saver when dealing with tight deadlines and massive codebases.
Regular Testing and Continuous Integration
We’ve all been guilty of pushing “just one more feature” without running the tests. But testing shouldn’t be an afterthought—it’s something you should do early and often. Implementing test-driven development (TDD) or integrating regular unit tests will prevent you from accidentally introducing bugs as your project scales.
Example: Simple Unit Tests for Critical Functions
Let’s say you’re developing a financial application, and you’ve written a function to calculate tax. Even a small bug in this function could have costly consequences. Write tests for it right away!
// Function to calculate tax
function calculateTax(amount) {
return amount * 0.2; // Fixed 20% tax
}
// Unit test for the tax function
test("calculates tax correctly", () => {
expect(calculateTax(100)).toBe(20);
expect(calculateTax(200)).toBe(40);
});
In the real world, regular testing can prevent an emergency at 2 a.m. when a critical function breaks after a deployment. Tools like Jest for JavaScript or PyTest for Python can automate this process, ensuring that bugs are caught before they go live.
Debugging with Precision
Debugging is part of every developer’s life, but it doesn’t have to be painful. Developing a precise and methodical debugging process can drastically reduce the time you spend searching for the root cause of an issue.
Real-World Scenario: Debugging a Production Issue
Imagine you’re maintaining an e-commerce site, and users are suddenly unable to place orders. Instead of aimlessly combing through the codebase, follow a structured process. Start by checking the logs.
# Look at the logs to pinpoint the issue
$ tail -f /var/log/app_errors.log
Once you’ve located the error, use strategic console logs or breakpoints to zero in on the problematic code. For instance, if your issue is related to an API call, logging the response status can give you immediate insight into what went wrong.
fetch("/api/orders")
.then((response) => {
console.log("API response status:", response.status);
return response.json();
})
.then((data) => console.log(data))
.catch((error) => console.error("API Error:", error));
Developing this methodical approach will save you countless hours of frustration, especially in high-stakes environments like production servers.
Continuous Learning and Adaptability
The tech landscape evolves quickly. Staying curious and embracing lifelong learning are essential for remaining competitive as a developer. Make it a habit to explore new technologies, frameworks, and methodologies—even if they don’t immediately apply to your current job.
Example: Learning a New JavaScript Framework
When I first decided to pick up Svelte, I was already comfortable with VueJs
. Instead of switching my entire project to Svelte right away, I started with a small demo app—like a simple to-do list. This allowed me to experiment with the framework’s features without feeling overwhelmed.
<script>
let tasks = [];
let newTask = "";
function addTask() {
if (newTask) {
tasks = [...tasks, newTask];
newTask = "";
}
}
</script>
<input
bind:value="{newTask}"
placeholder="Add a task"
/>
<button on:click="{addTask}">Add Task</button>
<ul>
{#each tasks as task}
<li>{task}</li>
{/each}
</ul>
This mindset of continuous learning will keep you agile in your career. Whether you’re transitioning to a new framework or learning cloud architecture, adaptability is a powerful asset.